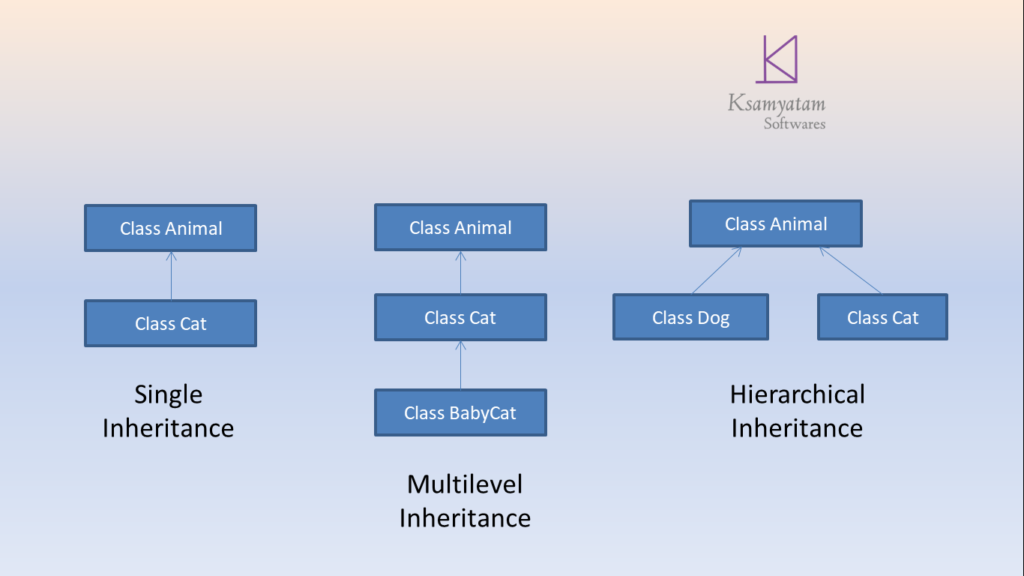
- Inheritance in Java is a process in which sub class inherits all the properties of super class(parent class).
- In Java, inheritance is an IS-A relationship (parent-child relationship).
- In Java, inheritance is an IS-A relationship (parent-child relationship).In Java, inheritance is used for Method Overriding ( Runtime Polymorphism).
- In Java, a sub class inherits is parent or superclass by using keyword “extends”.
Types of Inheritance in Java
1) Single
2) Multilevel
3) Hierarchical
4) Multiple(Multiple inheritance is not supported in Java through class)
5) Hybrid
Single Inheritance
public class Animal {
public void eat() {
System.out.println("Animal Eats Food");
}
}
public class Cat extends Animal{ // Single Inheritance
public void speak() {
System.out.println("Cat Says Meow");
}
}
Above example is called “Single Inheritance”, while below code represents “Multilevel Inheritance”.
public class BabyCat extends Cat{ // Multilevel Inheritance
public void sleep() {
System.out.println("Baby Cat is Sleeping");
}
}
Above Cat extends Animal represents Single Inheritance, while BabyCat extends Cat represents Multilevel Inheritance
public class InheritanceExample extends BabyCat{
// Example of Method Overriding
public void eat() {
System.out.println("Cat drinks Milk");
}
public static void main(String args[]) {
// Inheritance
BabyCat bCat = new BabyCat();
bCat.eat();
bCat.speak();
bCat.sleep();
// Inheritance And Method Overriding
InheritanceExample in_example = new InheritanceExample();
in_example.eat(); // Method Overriding
}
}
Output:
Animal Eats Food
Cat Says Meow
Baby Cat is Sleeping
Cat drinks Milk
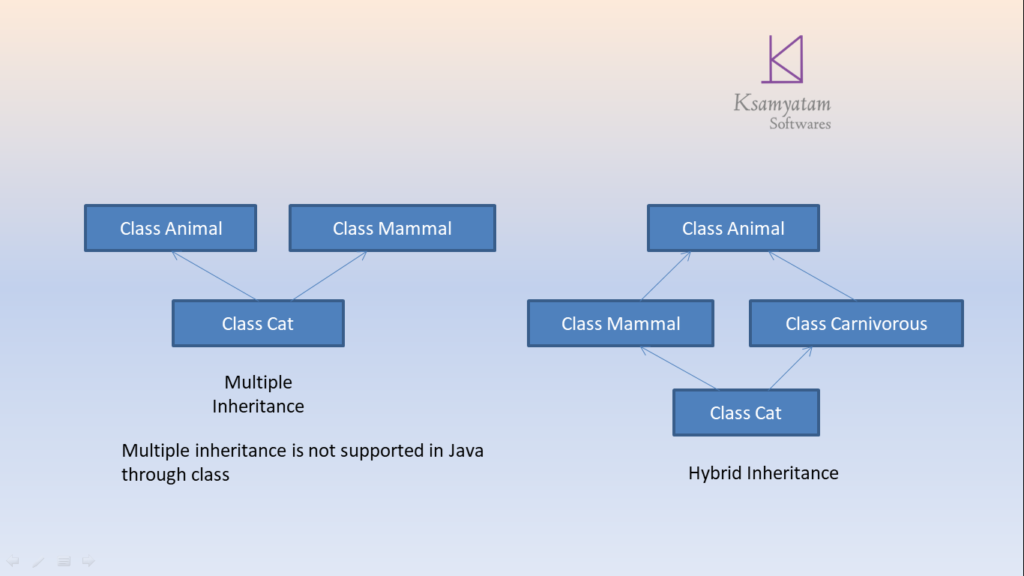