Java Method
Method in Java is a block of code which is used to execute specific tasks for which it is written and return the result to the caller.A Java method can perform some specific task without returning anything.It is used for code reusability and also increases code readability. It also allows to break program into smaller chunks of code.We write method once and can use it multiple times.Method in Java is executed only when we call or invoke it.For example, if we’ve written method to calculate area of circle then it will perform that task.
Advantage of Method
- Java method helps in code reusability
- Java method also helps in optimization of code
Syntax of Method :
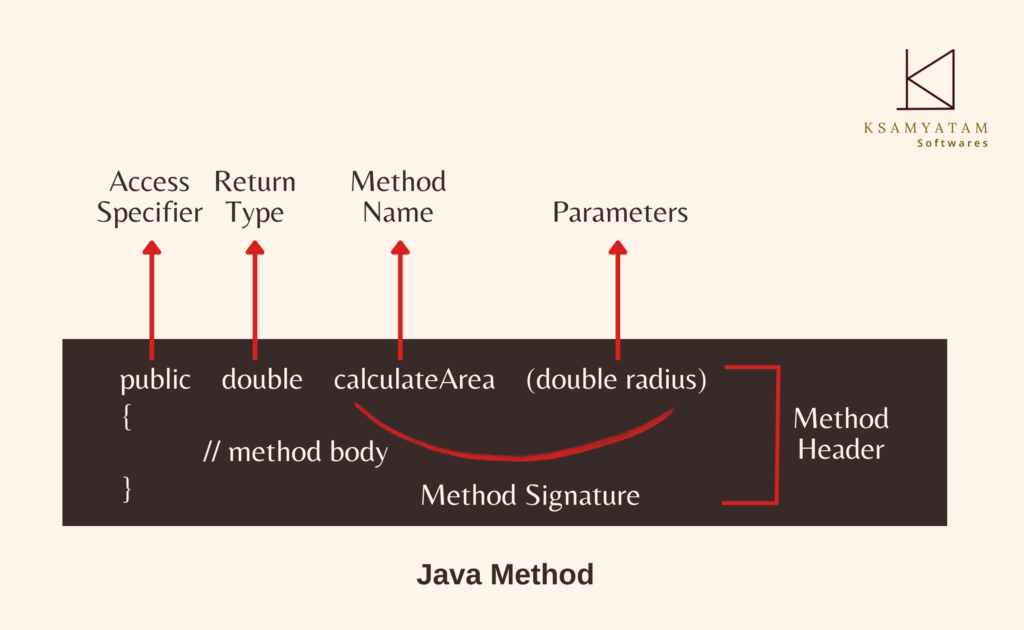
Method Declaration :
In Java method declarations have 6 component as follows :
1) Method Signature: Every method has a method signature which includes the method name and parameter list.
2) Access Specifier: Access specifier also called Access modifier is the access type of the method specifing the method visibility.In simple terms from where it can be accessed in your application.In Java, there 4 types of access specifiers.
Private: Access of a private modifier is only within the class and cannot be accessed from outside the class.
Default: Access of a default modifier is only within the package and cannot be accessed from outside the package. If no access modifier is specify then it will be default
Protected: Access of a protected modifier is within the package and outside the package through child class.
Public: Public access modifier can be accessed everywhere. It can be accessed from within and outside the class, within and outside the package.
3) Return Type: Return type is a data type which the method returns. Return type can be primitive data type, object, collection,etc. If the Java method does not return any value, we use void keyword with method.
4) Method Name: It is a name used to define the name of a method. Rules for field names apply to method names as well, but the convention is a little different. It must be relevant to the functionality of the method. Let say we write a method for addition of two numbers, the method name must be addition(). Java method is invoked by its name.
How to Name a Method?
A method name is typically a single word that should be a verb in lowercase or a multi-word, that begins with a verb in lowercase followed by an adjective, noun. After the first word, the first letter of each word should be capitalized.
Rules to Name a Method
While defining a method, remember that the method name must be a verb and start with a lowercase letter.
If the method name has more than two words, the first name must be a verb followed by an adjective or noun.
In the multi-word method name, the first letter of each word must be in uppercase except the first word. For example, doMultiply, computeMax, setX, and getX.
Generally, a method has a unique name within the class in which it is defined but sometimes a method might have the same name as other method names within the same class as method overloading is allowed in Java.
5) Parameter List: Parameter list contains the data type and variable name. It comma-separated and enclosed in the parentheses.If the method has no parameter, keep the parentheses blank.
6) Method Body: It is enclosed within the pair of curly braces. It contains the code that need to be executed to perform the intended operations and result
Types of Methods in Java :
There are two types of methods in Java:
- Predefined Method
- User-defined Method
- Predefined Method
In Java, predefined methods are the method that is already defined in the Java class libraries is known as predefined methods. It is also known as the standard library method or built-in method. We can directly use these methods just by calling them in the program at any point.Some pre-defined methods are length(), equals(), compareTo(), sqrt(), etc.Each and every predefined method is defined inside a class. Such as print() method is defined in the java.io.PrintStream class.
public class Demo
{
public static void main(String[] args)
{
// using the print() of PrintStream class and sqrt of Math class
System.out.print("The square root is: " + Math.sqrt(36));
}
}
Output:
The square root is: 6.0
- User-defined Method
The method written by the user or programmer is known as a user-defined method. These methods are modified according to the requirement.
Creating a User-defined Method :
Let’s create a user defined method that checks the number is even or odd. First, we will define the method.
//user defined method
public static void checkEvenOdd(int num)
{
//method body
if(num%2==0)
System.out.println(num+" is even");
else
System.out.println(num+" is odd");
}
We have defined the above method named checkEvenOdd(). It has a parameter num of data type int. Here the method does not return any value so keyword void is used. The method body contains the steps to check whether the number is even or odd. If the number is even, it prints the number is even, else prints the number is odd.
Calling Or Invoking a User-defined Method
After defining a method, it should be called. When we call or invoke a user-defined method, the program control gets transferred to the called method.
import java.util.Scanner;
public class EvenOdd
{
public static void main (String args[])
{
//creating an object of Scanner class
Scanner reader =new Scanner(System.in);
System.out.print("Enter the number: ");
//reading value that user entered
int num=reader.nextInt();
//method calling
checkEvenOdd(num);
}
}
In the above code snippet, as soon as the compiler reaches at line checkEvenOdd(num), the control gets transferred to the user-defined method and gives the output accordingly.
Above two snippets of codes can also be combined in a single program as shown below.
EvenOdd.java
import java.util.Scanner;
public class EvenOdd
{
public static void main (String args[])
{
//creating an object of Scanner class
Scanner reader =new Scanner(System.in);
System.out.print("Enter the number: ");
//reading value that user entered
int num=reader.nextInt();
//method calling
checkEvenOdd(num);
}
//user defined method
public static void checkEvenOdd(int num)
{
//method body
if(num%2==0)
System.out.println(num+" is even");
else
System.out.println(num+" is odd");
}
}
Output 1:
Enter the number: 10
10 is even
Output 2:
Enter the number: 45
45 is odd
Let’s see another program that return a value to the calling method.
In the following program, we have defined a method named multiple() that multiple the two numbers. It has two parameters n1 and n2 of integer type. The values of n1 and n2 correspond to the value of a and b, respectively. Therefore, the method adds the value of a and b and store it in the variable s and returns the sum.
Multiple.java
public class Multiple
{
public static void main(String[] args)
{
int a = 20;
int b = 4;
//method calling
int c = multiple(a, b); //a and b are actual parameters
System.out.println("The Multiplication of a and b is= " + c);
}
//user defined method
public static int multiple(int n1, int n2) //n1 and n2 are formal parameters
{
int value;
value=n1*n2;
return value; //returning the value
}
}
Output:
The Multiplication of a and b is= 80
- Instance Method:
Instance method is the method of the class which is a non-static method defined in the class. Before calling or invoking the instance method, it is mandatory to create an object of its class.
MultiplicationProgram.java
public class MultiplicationProgram
{
public static void main(String [] args)
{
//Creating an object of the class
MultiplicationProgram obj = new MultiplicationProgram();
//invoking instance method
System.out.println("The Multiplication is: "+obj.multiply(10, 4));
}
int value;
//user-defined method because we have not used static keyword
public int multiply(int a, int b)
{
value = a*b;
//returning the sum
return value;
}
}
Output:
The Multiplication is: 40
There are two types of instance method:
Accessor Method
Mutator Method
Accessor Method:
The method(s) that reads the instance variable(s) is known as the accessor method. We can easily identify it because the method is prefixed with the word get. It is also known as getters. It returns the value of the private field. It is used to get the value of the private field.
Example
public int getRollNumber()
{
return RollNumber;
}
Mutator Method:
The method(s) read the instance variable(s) and also modify the values. We can easily identify it because the method is prefixed with the word set. It is also known as setters or modifiers. It does not return anything. It accepts a parameter of the same data type that depends on the field. It is used to set the value of the private field.
Example
public void setRollNumber(int rollNumber)
{
this.rollNumber = rollNumber;
}
Example of accessor and mutator method
Student.java
public class Student
{
private int rollNumber;
private String studentName;
public int getRollNumber() {
return rollNumber;
}
public void setRollNumber(int rollNumber) {
this.rollNumber = rollNumber;
}
public String getStudentName() {
return studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public void display()
{
System.out.println("Roll no.: "+ rollNumber);
System.out.println("Student name: "+ studentName);
}
}
- Static Method:
A method that has static keyword is known as static method. In other words, a method that belongs to a class rather than an instance of a class is known as a static method. We can also create a static method by using the static keyword before the method name.
The main advantage of a static method is that we don’t have to create an object to call it. It can access static data members and also change its value. It is used to create an instance method. It is invoked by using the class name. main() method used in Java is a static method.
Example of static method
Student.java
public class Student
{
public static void main(String[] args)
{
examResult();
}
static void examResult()
{
System.out.println("Student Result Will Be Declared Soon");
}
}
Output:
Student Result Will Be Declared Soon
Abstract Method
The method that does not has method body is known as abstract method. In other words, Abstract methods are those types of methods that don’t require implementation for its declaration. Abstract method is declared only in the abstract class. To create an abstract method, we use the keyword abstract.
Syntax
abstract void method_name();
Example of abstract method
Result.java
abstract class Result //abstract class
{
//abstract method declaration
public abstract void display();
}
Student.java
public class Student extends Result
{
//method impelmentation
public void display()
{
System.out.println("Students Result Will Be Declared Soon");
}
public static void main(String args[])
{
//creating object of abstract class
Result obj = new Student();
//invoking abstract method
obj.display();
}
}
Output:
Students Result Will Be Declared Soon
Factory method
It is a method that returns an object to the class to which it belongs. All static methods are factory methods. For example,
NumberFormat number = NumberFormat.getNumberInstance();
String str_value = String.valueOf('a');